轉接器
轉接器是一種伺服器端元件,負責將事件廣播給所有或部分用戶端。
當擴充到多個 Socket.IO 伺服器時,您需要以其他實作取代預設的記憶體內轉接器,以便將事件正確路由到所有用戶端。
以下是我們團隊維護的轉接器清單
- the Redis 轉接器
- the Redis Streams 轉接器
- the MongoDB 轉接器
- the Postgres 轉接器
- the 叢集轉接器
- the Google Cloud Pub/Sub 轉接器
- the AWS SQS 轉接器
- the Azure Service Bus 轉接器
還有其他幾個選項由(很棒的!)社群維護
請注意,在使用多個 Socket.IO 伺服器和 HTTP 長輪詢時,仍需要啟用黏著式工作階段。更多資訊 在此。
API
您可以透過以下方式存取適配器實例
// main namespace
const mainAdapter = io.of("/").adapter; // WARNING! io.adapter() will not work
// custom namespace
const adminAdapter = io.of("/admin").adapter;
從 socket.io@3.1.0
開始,每個適配器實例都會發出下列事件
create-room
(參數:房間)delete-room
(參數:房間)join-room
(參數:房間、ID)leave-room
(參數:房間、ID)
範例
io.of("/").adapter.on("create-room", (room) => {
console.log(`room ${room} was created`);
});
io.of("/").adapter.on("join-room", (room, id) => {
console.log(`socket ${id} has joined room ${room}`);
});
發射器
大多數適配器實作都附帶相關的發射器套件,可讓您從另一個 Node.js 程序與 Socket.IO 伺服器群組進行通訊。
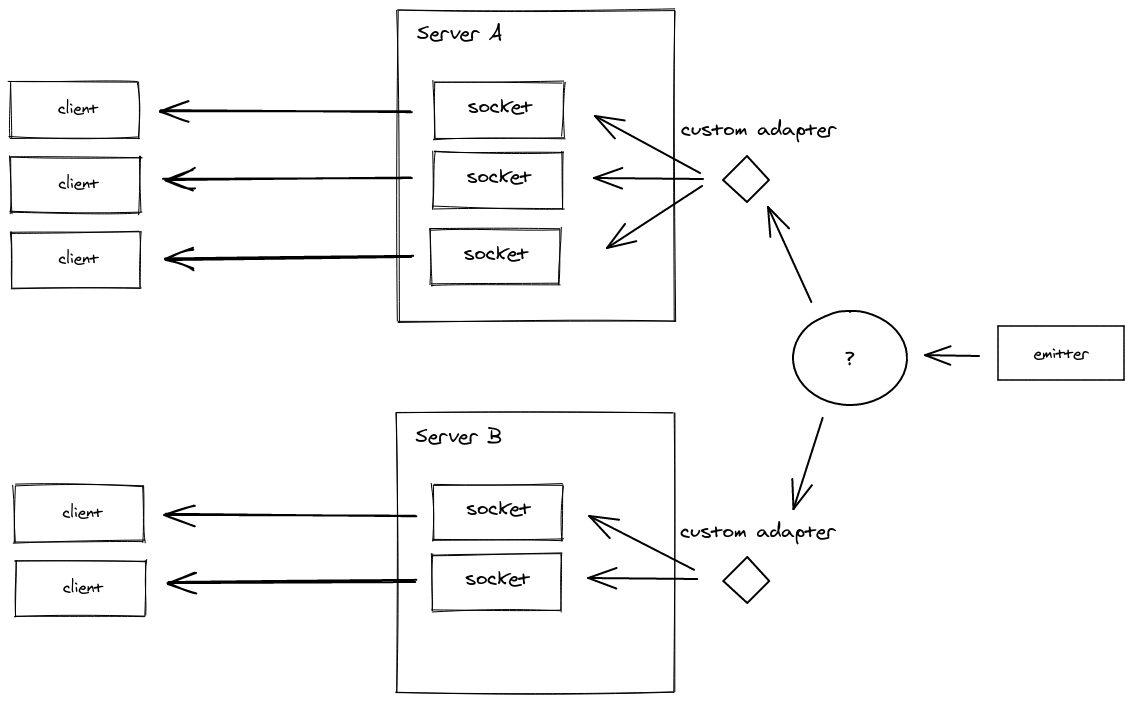
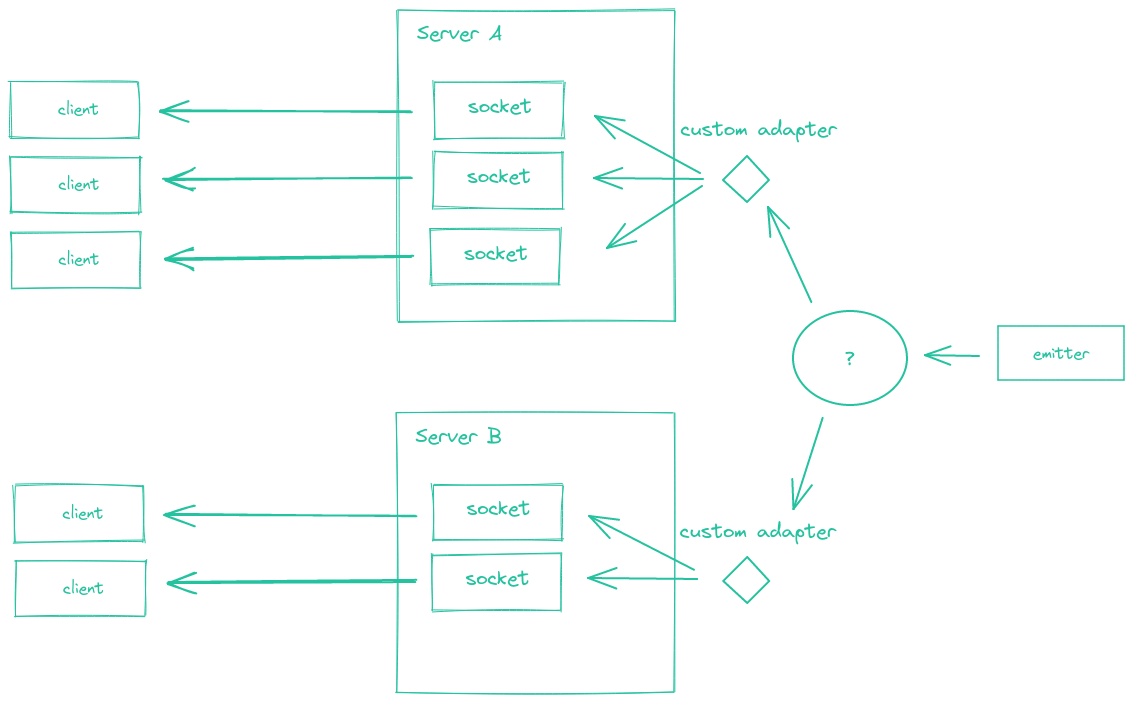
這在微服務設定中可能很有用,其中所有用戶端都連線到微服務 M1,而微服務 M2 則使用發射器廣播封包(單向通訊)。
發射器秘笈
// to all clients
emitter.emit(/* ... */);
// to all clients in "room1"
emitter.to("room1").emit(/* ... */);
// to all clients in "room1" except those in "room2"
emitter.to("room1").except("room2").emit(/* ... */);
const adminEmitter = emitter.of("/admin");
// to all clients in the "admin" namespace
adminEmitter.emit(/* ... */);
// to all clients in the "admin" namespace and in the "room1" room
adminEmitter.to("room1").emit(/* ... */);
發射器也支援在 socket.io@4.0.0
中新增的公用程式方法
socketsJoin()
// make all Socket instances join the "room1" room
emitter.socketsJoin("room1");
// make all Socket instances of the "admin" namespace in the "room1" room join the "room2" room
emitter.of("/admin").in("room1").socketsJoin("room2");
socketsLeave()
// make all Socket instances leave the "room1" room
emitter.socketsLeave("room1");
// make all Socket instances in the "room1" room leave the "room2" and "room3" rooms
emitter.in("room1").socketsLeave(["room2", "room3"]);
// make all Socket instances in the "room1" room of the "admin" namespace leave the "room2" room
emitter.of("/admin").in("room1").socketsLeave("room2");
disconnectSockets()
// make all Socket instances disconnect
emitter.disconnectSockets();
// make all Socket instances in the "room1" room disconnect (and discard the low-level connection)
emitter.in("room1").disconnectSockets(true);
// make all Socket instances in the "room1" room of the "admin" namespace disconnect
emitter.of("/admin").in("room1").disconnectSockets();
// this also works with a single socket ID
emitter.of("/admin").in(theSocketId).disconnectSockets();
serverSideEmit()
// emit an event to all the Socket.IO servers of the cluster
emitter.serverSideEmit("hello", "world");
// Socket.IO server (server-side)
io.on("hello", (arg) => {
console.log(arg); // prints "world"
});